Freedom of assembly
Assembly language is closer to what a computer understands and less
like what a human would understand. but it is fast and compact. when
assembled and linked vs a compiled higher level program. Assembly
language version of Hello world:
or
No real lesson here except to expose you to how it looks. To make it a program where the preceding code was saved as hw.asm, you would:
$ nasm -f elf hw.asm
$ ld -s -o hw hw.o
$ ./hw
Hello world!
~$ ls -alh hw
-rwxrwxr-x 1 eddie eddie 360 Sep 19 02:09 hw
The same program in the C language without comments that would really make it a larger program of source code.
Save file as “hello.c”. To make this program, you would:
$ gcc hw.c -o hw
$ ./hw
Hello world!
$ ls -alh hw
-rwxrwxr-x 1 eddie eddie 7.0K Sep 19 02:12 hw
The hw.c file creates a program file of 8298 ot less bytes. the hw.asm file creates a program file of 440 or less bytes. You can see that though the assembly program is a bit longer it generates a much smaller file. So assembly programs being all things are equal could save you up to ninety or more percent disk space. If you are renting storage, this could be a real money saver. Also the increase of speed in your system saves time and money also in other ways.
————————————————————————
Other examples of hello world:
Cobol (open-cobol using free form) Known for being wordy, but it is also self documenting.
Basic (freebasic)
$ fbc -lang qb hw.bas
$ ./hw
Hello world!
Pascal (free pascal)
$ fpc hw.pas
Free Pascal Compiler version 2.4.4-3.1 [2012/01/04] for i386
Copyright (c) 1993-2010 by Florian Klaempfl
Target OS: Linux for i386
Compiling hw.pas
Linking hw
/usr/bin/ld: warning: link.res contains output sections; did you forget -T?
4 lines compiled, 0.2 sec
$ ./hw
Hello World!
C++ (g++) New age version of C?
Java (javac java)
Save file as “HelloWorld.java”. To make this program, you would:
Python (interpreted)
Python 2.6.5 (r265:79063, Apr 16 2010, 13:09:56)
[GCC 4.4.3] on linux2
Type “help”, “copyright”, “credits” or “license” for more information.
>>> print “Hello World!”
Hello World!
>>> exit()
or
#!/usr/bin/python
# start
print “Hello World!”;
# end
Save file as “hw.py”. To make this program, you would:
$ python hw.py
Hello World!
Bash (interpreted)
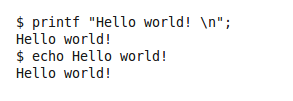
Fortran (gfortran (do not forget to start at column 6))
section .data hello: db 'Hello world!',10 ; 'Hello world!' plus a linefeed character helloLen: equ $-hello ; Length of the 'Hello world!' string ; (I'll explain soon) section .text global _start _start: mov eax,4 ; The system call for write (sys_write) mov ebx,1 ; File descriptor 1 - standard output mov ecx,hello ; Put the offset of hello in ecx mov edx,helloLen ; helloLen is a constant, so we don't need to say ; mov edx,[helloLen] to get it's actual value int 80h ; Call the kernel mov eax,1 ; The system call for exit (sys_exit) mov ebx,0 ; Exit with return code of 0 (no error) int 80h
or
No real lesson here except to expose you to how it looks. To make it a program where the preceding code was saved as hw.asm, you would:
$ nasm -f elf hw.asm
$ ld -s -o hw hw.o
$ ./hw
Hello world!
~$ ls -alh hw
-rwxrwxr-x 1 eddie eddie 360 Sep 19 02:09 hw
The same program in the C language without comments that would really make it a larger program of source code.
#include <stdio.h> main() { printf ("Hello world!\n"); }
Save file as “hello.c”. To make this program, you would:
$ gcc hw.c -o hw
$ ./hw
Hello world!
$ ls -alh hw
-rwxrwxr-x 1 eddie eddie 7.0K Sep 19 02:12 hw
The hw.c file creates a program file of 8298 ot less bytes. the hw.asm file creates a program file of 440 or less bytes. You can see that though the assembly program is a bit longer it generates a much smaller file. So assembly programs being all things are equal could save you up to ninety or more percent disk space. If you are renting storage, this could be a real money saver. Also the increase of speed in your system saves time and money also in other ways.
————————————————————————
Other examples of hello world:
Cobol (open-cobol using free form) Known for being wordy, but it is also self documenting.
IDENTIFICATION DIVISION. PROGRAM-ID. hello. PROCEDURE DIVISION. DISPLAY "Hello World!". STOP RUN.
Save file as “hello.cob”. To make this program, you would:
$ cobc -O -x -free hello.cob $ ./hello Hello, world!
or more primitive form (do not forget to skip seven spaces)
* Sample COBOL program IDENTIFICATION DIVISION. PROGRAM-ID. hello. PROCEDURE DIVISION. DISPLAY "Hello World!". STOP RUN.
The compiler is cobc, which is executed as follows:
$ cobc -O -x hw.cob $ ./hello Hello World!
Basic (freebasic)
'rem start
print"Hello world!" end
Save file as “hello.bas”. To make this program, you would:
$ fbc -lang qb hw.bas
$ ./hw
Hello world!
Pascal (free pascal)
program Hello_World; Begin writeln('Hello World!') End.
Save file as “hw.pas”. To make this program, you would: (ignore error)
$ fpc hw.pas
Free Pascal Compiler version 2.4.4-3.1 [2012/01/04] for i386
Copyright (c) 1993-2010 by Florian Klaempfl
Target OS: Linux for i386
Compiling hw.pas
Linking hw
/usr/bin/ld: warning: link.res contains output sections; did you forget -T?
4 lines compiled, 0.2 sec
$ ./hw
Hello World!
C++ (g++) New age version of C?
#include <iostream> using namespace std; //hello.cpp int main() { cout << "Hello world!\n"; return 0; }
Save file as “hello.cpp”. To make this program, you would:
$ g++ hw.cpp -o hw $ ./hw Hello world!
Java (javac java)
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World"); } }
$ javac HelloWorld.java
$ java HelloWorld
Hello, World
$ java HelloWorld
Hello, World
print "Hello World!" exit()$ python
Python 2.6.5 (r265:79063, Apr 16 2010, 13:09:56)
[GCC 4.4.3] on linux2
Type “help”, “copyright”, “credits” or “license” for more information.
>>> print “Hello World!”
Hello World!
>>> exit()
or
#!/usr/bin/python
# start
print “Hello World!”;
# end
Save file as “hw.py”. To make this program, you would:
$ python hw.py
Hello World!
Bash (interpreted)
$ printf "Hello world! \n";
$ echo Hello world!
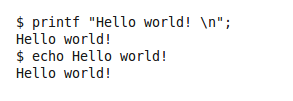
Fortran (gfortran (do not forget to start at column 6))
program helloworld print *,"Hello World!" end program helloworld
Save file as “hw.for”. To make this program, you would:
$ gfortran hw.for $ ./hw Hello world!
Anyway, just some examples of “Hello world!” code to wet your appetite for programming. Notice any similarities/differences in all this. All the examples worked “as is” on linux with free software.
Dom Of Assembly >>>>> Download Now
ReplyDelete>>>>> Download Full
Dom Of Assembly >>>>> Download LINK
>>>>> Download Now
Dom Of Assembly >>>>> Download Full
>>>>> Download LINK GV